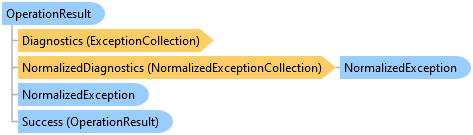
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult)> <ComVisibleAttribute(True)> <GuidAttribute("8708B3B9-7BA4-4E9B-A2A7-4AC5CA7CE1AE")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class OperationResult Inherits OpcLabs.BaseLib.Info Implements OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As OperationResult
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult)] [ComVisible(true)] [Guid("8708B3B9-7BA4-4E9B-A2A7-4AC5CA7CE1AE")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class OperationResult : OpcLabs.BaseLib.Info, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult)] [ComVisible(true)] [Guid("8708B3B9-7BA4-4E9B-A2A7-4AC5CA7CE1AE")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class OperationResult : public OpcLabs.BaseLib.Info, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
The result is successful if the Exception is a null reference. Otherwise, this property contains information about the reason of the failure.
The OperationResult is a base class that does not contain any value for successful results. The derived classes add properties that contain such values.
The OperationResult class is a fundamental part of the operation model, designed to provide a consistent way to represent the outcomes of operations across different layers of an application. It includes support for:
// This example shows how to write values into 3 items at once, test for success of each write and display the exception // message in case of failure. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Diagnostics; using OpcLabs.BaseLib.OperationModel; using OpcLabs.EasyOpc.DataAccess; using OpcLabs.EasyOpc.DataAccess.OperationModel; namespace DocExamples.DataAccess._EasyDAClient { partial class WriteMultipleItemValues { public static void TestSuccess() { // Instantiate the client object. var client = new EasyDAClient(); Console.WriteLine("Writing multiple item values..."); OperationResult[] resultArray = client.WriteMultipleItemValues( new[] { new DAItemValueArguments("", "OPCLabs.KitServer.2", "Simulation.Register_I4", 23456), new DAItemValueArguments("", "OPCLabs.KitServer.2", "Simulation.Register_R8", "This string cannot be converted to VT_R8"), new DAItemValueArguments("", "OPCLabs.KitServer.2", "UnknownItem", "ABC") }); for (int i = 0; i < resultArray.Length; i++) { Debug.Assert(resultArray[i] != null); if (resultArray[i].Succeeded) Console.WriteLine("Result {0}: success", i); else { Debug.Assert(!(resultArray[i].Exception is null)); Console.WriteLine("Result {0} *** Failure: {1}", i, resultArray[i].ErrorMessageBrief); } } } // Example output: // //Writing multiple item values... //Result 0: success //Result 1 *** Failure: Type mismatch. [...] //Result 2 *** Failure: The item is no longer available in the server address space. [...] } }
' This example shows how to write values into 3 items at once, test for success of each write and display the exception ' message in case of failure. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.BaseLib.OperationModel Imports OpcLabs.EasyOpc.DataAccess Imports OpcLabs.EasyOpc.DataAccess.OperationModel Namespace DataAccess._EasyDAClient Partial Friend Class WriteMultipleItemValues Public Shared Sub TestSuccess() ' Instantiate the client object. Dim client = New EasyDAClient() Console.WriteLine("Writing multiple item values...") Dim resultArray As OperationResult() = client.WriteMultipleItemValues(New DAItemValueArguments() { New DAItemValueArguments("", "OPCLabs.KitServer.2", "Simulation.Register_I4", 23456), New DAItemValueArguments("", "OPCLabs.KitServer.2", "Simulation.Register_R8", "This string cannot be converted to VT_R8"), New DAItemValueArguments("", "OPCLabs.KitServer.2", "UnknownItem", "ABC") }) For i = 0 To resultArray.Length - 1 Debug.Assert(resultArray(i) IsNot Nothing) If resultArray(i).Succeeded Then Console.WriteLine("Result {0}: success", i) Else Debug.Assert(resultArray(i).Exception IsNot Nothing) Console.WriteLine("Result {0} *** Failure: {1}", i, resultArray(i).ErrorMessageBrief) End If Next i End Sub ' Example output ' 'Writing multiple item values... 'Result 0: success 'Result 1 *** Failure: Type mismatch. [...] 'Result 2 *** Failure: The item Is no longer available In the server address space. [...] End Class End Namespace
// This example shows how to write values into 3 nodes at once, test for success of each write and display the exception // message in case of failure. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.BaseLib.OperationModel; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples._EasyUAClient { partial class WriteMultipleValues { public static void TestSuccess() { UAEndpointDescriptor endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; // or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) // or "https://opcua.demo-this.com:51212/UA/SampleServer/" // Instantiate the client object. var client = new EasyUAClient(); Console.WriteLine("Modifying values of nodes..."); OperationResult[] operationResultArray = client.WriteMultipleValues(new[] { new UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10221", 23456), new UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10226", "This string cannot be converted to Double"), new UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;s=UnknownNode", "ABC") }); for (int i = 0; i < operationResultArray.Length; i++) if (operationResultArray[i].Succeeded) Console.WriteLine($"Result {i}: success"); else Console.WriteLine($"Result {i}: {operationResultArray[i].Exception.GetBaseException().Message}"); // Example output: // //Modifying values of nodes... //Result 0: success //Result 1: Input string was not in a correct format. //+ Attempting to change an object of type "System.String" to type "System.Double". //+ The specified original value (string) was "This string cannot be converted to Double". //+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;i=10226". //+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'. //Result 2: The status of the OPC-UA attribute data is not Good. The actual status is 'BadNodeIdUnknown'. //+ During writing or method calls, readings may occur when value type is not specified. //+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;s=UnknownNode". //+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'. } } }
# This example shows how to write values into 3 nodes at once, test for success of each write and display the exception # message in case of failure. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. #requires -Version 5.1 using namespace OpcLabs.EasyOpc.UA using namespace OpcLabs.EasyOpc.UA.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUA.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUAComponents.dll" [UAEndpointDescriptor]$endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" # or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) # or "https://opcua.demo-this.com:51212/UA/SampleServer/" # Instantiate the client object. $client = New-Object EasyUAClient Write-Host "Modifying values of nodes..." $operationResultArray = $client.WriteMultipleValues([UAWriteValueArguments[]]@( (New-Object UAWriteValueArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10221", 23456)), (New-Object UAWriteValueArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10226", "This string cannot be converted to Double")), (New-Object UAWriteValueArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;s=UnknownNode", "ABC")) )) for ($i = 0; $i -lt $operationResultArray.Length; $i++) { if ($operationResultArray[$i].Succeeded) { Write-Host "Result $($i): success" } else { Write-Host "Result $($i): $($operationResultArray[$i].Exception.GetBaseException().Message)" } } # Example output: # #Modifying values of nodes... #Result 0: success #Result 1: Input string was not in a correct format. #+ Attempting to change an object of type "System.String" to type "System.Double". #+ The specified original value (string) was "This string cannot be converted to Double". #+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;i=10226". #+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'. #Result 2: The status of the OPC-UA attribute data is not Good. The actual status is 'BadNodeIdUnknown'. #+ During writing or method calls, readings may occur when value type is not specified. #+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;s=UnknownNode". #+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'.
' This example shows how to write values into 3 nodes at once, test for success of each write and display the exception ' message in case of failure. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports System Imports OpcLabs.BaseLib.OperationModel Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.OperationModel Namespace _EasyUAClient Partial Friend Class WriteMultipleValues Public Shared Sub TestSuccess() ' Define which server we will work with. Dim endpointDescriptor As UAEndpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" ' or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) ' or "https://opcua.demo-this.com:51212/UA/SampleServer/" ' Instantiate the client object Dim client = New EasyUAClient() ' Modify value of a node Dim operationResultArray() As OperationResult = client.WriteMultipleValues(New UAWriteValueArguments() _ { _ New UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10221", 23456), _ New UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10226", "This string cannot be converted to Double"), _ New UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;s=UnknownNode", "ABC") _ } _ ) For i As Integer = 0 To operationResultArray.Length - 1 If operationResultArray(i).Succeeded Then Console.WriteLine("Result {0}: success", i) Else Console.WriteLine("Result {0}: {1}", i, operationResultArray(i).Exception.GetBaseException().Message) End If Next i End Sub End Class End Namespace
# This example shows how to write values into 3 nodes at once, test for success of each write and display the exception # message in case of failure. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.OperationModel import * endpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:51210/UA/SampleServer') # or 'http://opcua.demo-this.com:51211/UA/SampleServer' (currently not supported) # or 'https://opcua.demo-this.com:51212/UA/SampleServer/' # Instantiate the client object client = EasyUAClient() print('Modifying values of nodes...') writeResultArray = IEasyUAClientExtension.WriteMultipleValues(client, [ UAWriteValueArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10221'), 23456), UAWriteValueArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10226'), 'This string cannot be converted to Double'), UAWriteValueArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;s=UnknownNode'), 'ABC'), ]) for i, writeResult in enumerate(writeResultArray): if writeResult.Succeeded: print('writeResultArray[', i, ']: success', sep='') else: print('writeResultArray[', i, '] *** Failure: ', writeResult.Exception.GetBaseException().Message, sep='') print() print('Finished.')
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.OperationModel.OperationResult
OpcLabs.BaseLib.OperationModel.ValueArrayResult
OpcLabs.BaseLib.OperationModel.ValueResult
OpcLabs.EasyOpc.DataAccess.OperationModel.DAVtqResult
OpcLabs.EasyOpc.UA.OperationModel.UAAttributeDataResult
OpcLabs.EasyOpc.UA.OperationModel.UAWriteResult